Event listeners are custom functions that are invoked whenever some action or event, like for instance an object is clicked, or series is hidden, or axis is zoomed, is triggered.
Attaching events
Every object in amCharts 4 has a property events
which is an "event dispatcher".
Event dispatcher is responsible for registering and de-registering custom functions, as well as executing them whenever certain event occurs in the dispatchers parent object.
To register your function to be executed whenever something happens, you use events.on()
or events.once()
.
The difference between the two is that the former will make sure function is called every time an event of that type occurs, while the latter will execute only on the first occurrence.
Both on()
and once()
take three parameters:
# | Parameter | Type | Comment |
---|---|---|---|
1 | type | string |
A specific event indicator like "hidden", "propertychanged", etc. |
2 | callback | function |
A callback function that will be called whenever event occurs. |
3 | scope (optional) | any |
A scope in which callback function will be executed. |
Here's an example that attaches click event to a column in a Column series:
series.columns.template.events.on("hit", function(ev) { console.log("clicked on ", ev.target); }, this);
series.columns.template.events.on("hit", function(ev) { console.log("clicked on ", ev.target); }, this);
{ // ... "series": [{ "type": "ColumnSeries", // ... "events": { "hit": function(ev) { console.log("clicked on ", ev.target); } } }] }
NOTE amCharts 4 uses "hit" event to indicated universal click or touch event.
Available events
For available events for each individual object look up "Events" section in that class' reference.
For example check out Column series events.
The table will show the event identificator (type), e.g. "hit" as well as the precise structure of the event object that will be passed into your custom function whenever event occurs.
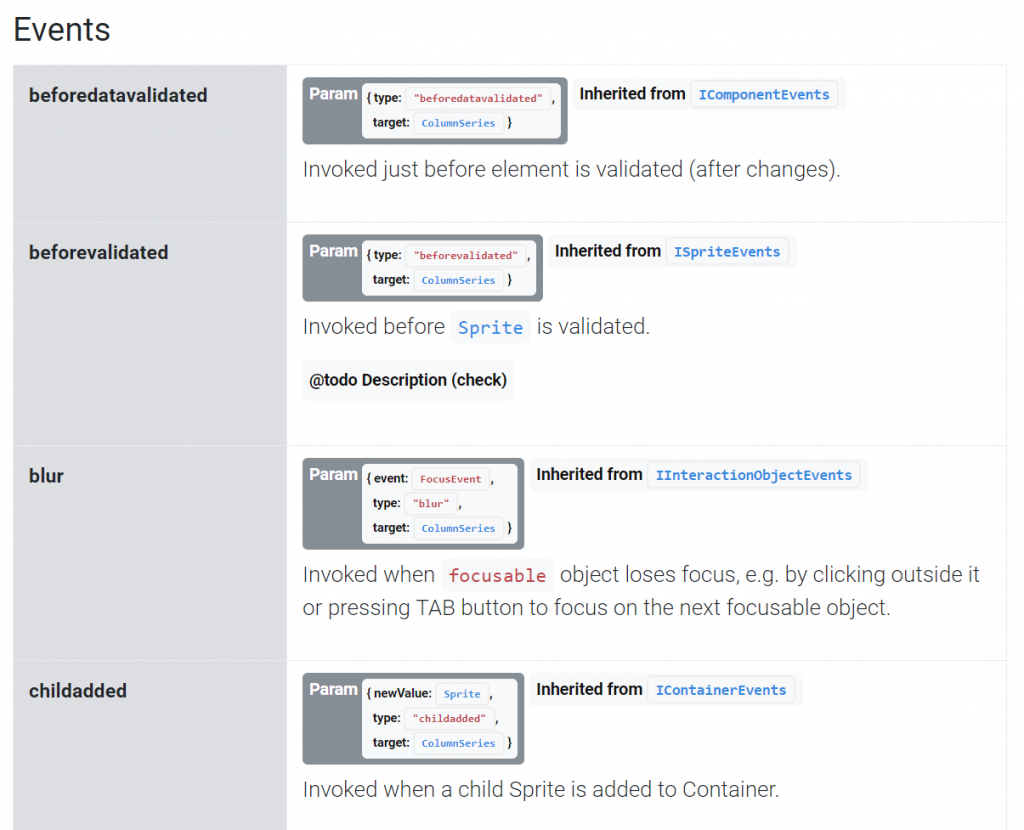
Removing events
There are couple of ways to remove existing events.
Using off() function
To remove specific event, simply use events.off()
with the same parameter you used to add event.
Please note, to remove event you need to pass in identical parameters, including the reference to the same callback function, and scope if any.
This means that it won't work for the previous example, since we have used an anonymous function.
If we wanted to add an event for later with an off()
function, we'd need to define a function, which we could reference in both calls:
function myFunction(ev) { console.log("clicked on ", ev.target); } series.columns.template.events.on("hit", myFunction, this); series.columns.template.events.off("hit", myFunction, this);
function myFunction(ev) { console.log("clicked on ", ev.target); } series.columns.template.events.on("hit", myFunction, this); series.columns.template.events.off("hit", myFunction, this);
Using disposers
Whenever an event is created, the on()
or off()
function returns a so called "disposer" object.
We can assign it to for safekeeping in a variable. Then, when we don't need the event anymore, simply call dispose()
method on it. That will remove event handler.
let myEvent = series.columns.template.events.on("hit", function(ev) { console.log("clicked on ", ev.target); }, this); myEvent.dispose();
var myEvent = series.columns.template.events.on("hit", function(ev) { console.log("clicked on ", ev.target); }, this); myEvent.dispose();
Disabling events
To temporarily disable event handler invocation for certain event type, use event dispatcher's disableType(type)
method.
For example, to temporarily disable all event handlers for "hit" event on columns in a series, you'd do this:
series.columns.template.events.disableType("hit");
series.columns.template.events.disableType("hit");
To re-enable, simply call enableType(type)
.
To disable all event handler activity for the object, use event dispatchers disable()
method.
// No event handlers will be called after this series.columns.template.events.disable();
// No event handlers will be called after this series.columns.template.events.disable();
As you may already guessed, to enable back event handlers, use enable()
method.
"Catch-all" handlers
It is possible, albeit not recommended, to set up a "catch all" event handlers.
Such handlers will be invoked on all events that occur in target object.
To add such handler, use event dispatcher's onAll()
method.
It works exactly like on()
with the exception that there's no event type to specify, so we're down to two parameters only:
series.columns.template.events.onAll(function(ev) { console.log("something happened ", ev); }, this);
series.columns.template.events.onAll(function(ev) { console.log("something happened ", ev); }, this);
Manually triggering events
Sometimes, you maybe need to trigger an event manually. Like for example you might want to trigger a hit event on a "zoom out" button on a chart, when something else happens in your page or application.
For just that case, each and every object, has two methods dispatchImmediately(event_type)
and dispatch(event_type)
.
Both will trigger an event, with the slight difference of that the latter will kick in immediately, while the later will wait for the next frame.
chart.zoomOutButton.dispatchImmediately("hit");
chart.zoomOutButton.dispatchImmediately("hit");